Authentication and Authorization
Authentication and authorization are vital aspects of API security. Therefore, every request made to BASF APIs must be properly authorized. BASF APIs feature state-of-the-art security to keep all interactions with your applications safe and confidential.
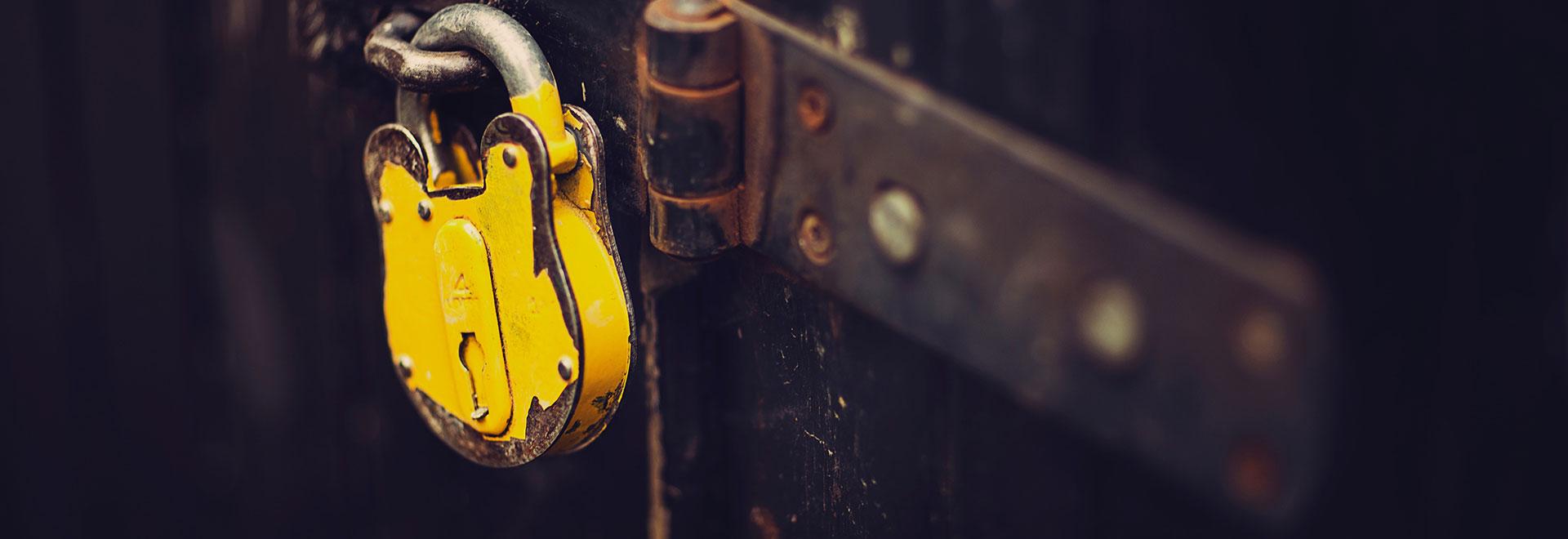
In this guide, you learn how authentication and authorization work and how to implement it.
Terminology
Authentication means establishing an identity, i.e., to find out whether someone is who they say they are. Both applications and users have an identity.
Authorization means being able to prove that someone is allowed to access a specific resource. Typically, authentication and authorization are part of the same workflow.
Before integrating any BASF APIs in your application, you need to register your application on the developer portal. The portal provides you a Client ID and a Client Secret. These are the credentials that your application uses to authenticate itself, i.e., to let the API know which client is accessing.
As you’re implementing authentication in your application, the essential information to determine is which of the two scenarios listed below applies to the API product that you’re integrating.
App-Only Context: For some BASF API products, it is sufficient to know the identity of the application. These APIs are also described as working in an app-only context.
App and User Context: Other API products also need to know the identity of the person who is using the application, on top of the application’s identity. These APIs are said to work in an app and user context.
Once you have gathered that information from the API’s documentation in the developer portal, please read the respective section.
App-Only Context
For BASF API products with an app-only context, there are two different approaches to authentication and authorization, API Keys and Functional Users.
API Keys
APIs that provide access to public data require an API key. This API key is equivalent to the Client ID of the application that you have registered.
There is no separate authentication step or workflow to execute before you can make authenticated API calls. You solely have to add the API key, or the Client ID, to every request you make.
The parameter is typically called x-api-key and could be a custom HTTP header or included in the query part of the URL. That is not standardized, so you should refer to the respective API product's documentation to find out how to send the API key.
Functional Users
APIs that provide access to confidential or sensitive information require a functional user. Unlike a personal user account, a functional user is a role account that doesn't belong to a specific individual. These users use client certificates to authenticate against a particular endpoint and subsequently use the OAuth protocol in a similar way as API products with an app and user context do.
Getting a Certificate
The BASF Group's API team can help you set up a functional user, create a private key, and issue a certificate. Write to developer@basf.com or use the contact form on the portal to learn more.
The OAuth Flow
The section about the App and User Context APIs contains a general introduction to OAuth as well as how BASF uses the protocol. Most of the steps are similar for functional users, therefore read those sections first to familiarize yourself with OAuth.
Afterward, move to the OAuth with Client Certificates section to learn how to implement the OAuth flow with certificates.
App and User Context
Every BASF API product with an app and user context requirement uses OAuth 2.0, a standardized, open protocol for secure authorization.
If you are familiar with OAuth, for example, because you have used APIs from other providers that also leverage the protocol, you can skip the OAuth Overview and move on to OAuth at BASF.
OAuth Overview
The following two sections provide an overview of OAuth, first explaining the user experience and then the technical details and terminology.
User Experience
For the end-user of an application, the OAuth flow appears as follows:
- Once the application needs to access an external API with the context of the current user, it requests this authorization from the user. Typically, the application does this by sending the user to a specific web page in their browser. This page is hosted by the entity that offers the API (the “API Provider”).
- The user interacts with the page to authenticate themselves and give the proper authorization for the application. The design and flow of this process is solely the API Provider’s responsibility.
- After the user interaction is complete, the page sends them back to the application.
As a result of this workflow, the client application receives a unique, typically time-limited credential. This credential has to be sent with every further API request and encapsulates both app and user context.
Protocol Details
From a technical perspective, an OAuth flow involves four parties. Following the terminology of the protocol specification, they are called client, resource owner, authorization server, and resource server:
- The client, e.g., the application consuming an API, sends an authorization request to the resource owner, e.g., the current user. This request produces an authorization grant.
- Next, the client takes this authorization grant and sends it to the authorization server. That server replies with an access token.
- Finally, the client can use the access token to make requests to the resource server and access the protected resources.
OAuth is a framework that covers various authorization scenarios. Thus, there are multiple types of authorization grants. The most common type is the authorization code grant.
These are the steps for the authorization grant workflow that a client needs to implement:
- Take the authorization endpoint URL from the API provider, add the required query parameters, and send the user to this URL. Web-based applications can do a simple redirect, whereas native applications on mobile or desktop need to open an external browser or show an embedded browser. You don’t need to care through which actions the authorization endpoint leads the user to establish their identity and authorize the application; you can wait until the user returns. The authorization server adds a code parameter to the URL used to redirect the user back.
- From your application – on the server-side if possible – make an HTTP POST request to the token endpoint URL from the API provider, authenticating yourself (as an application) with the Client ID and Client Secret and sending the code parameter. If the grant is accepted, you receive an access token back.
- Store the access token and make API requests with it.
Some token endpoints send a refresh token together with the access token. In this case, the access token is typically short-lived, but you can retrieve a new access token without needing to send the user through the authorization endpoint again. You can send the refresh token to the token endpoint together with your Client ID and Client Secret to retrieve a new access token, no user interaction required.
OAuth at BASF
This section describes the endpoints and parameters required for implementing an OAuth flow for BASF APIs.
BASF exclusively uses the authorization code grant. All access tokens are valid only for a limited time, after which you have to reauthorize. It is possible to extend the authorization with a refresh token. However, whether you receive a refresh token or not depends on the configuration of your application, the API products that you consume, and the scope that you provided for them. Hence your application should be able to handle responses from the authorization endpoint both with and without refresh tokens.
Authorization Endpoint
The endpoint to which you send your users for authentication is located at the following URL:
https://prod.api.basf.com/security/internal/v1/oauth2/login
While building the URL, you need to add the following query parameters:
Name |
Description |
Example |
Notes |
---|---|---|---|
response_type |
The type of response expected from the endpoint. Must be “code”. |
code |
We use the “code” grant type exclusively. |
redirect_uri |
URL to send the user to after authentication. |
https://example.com/ |
This parameter must match the URL provided during application registration. |
scope |
One or more scopes which you want to request. Separate multiple scopes with spaces. |
apim |
Use openid to get user identity information. Other applicable scopes as found in the respective API documentation. |
client_id |
Your application’s Client ID |
c653ff6e-a86a-4531-8443-a8a4378dc2fb |
The Client ID for your application can be found under My Apps in the developer portal. |
Please note that the state parameter is currently not supported.
On the authorization endpoint, users have the following options to authenticate against NetIQ, the centralized identity provider of BASF:
- A browser client certificate with the private key on their BASF smartcard. This is the preferred option for company users.
- A mobile OTP.
- A hardware RSA token.
- A username and password. This option is only available when the user is within the BASF private network.
The authentication method has no relevance for the development of your client application, as the whole process happens in the browser, between the user and the identity provider.
After the user has authenticated, the identity provider redirects them to your redirect_uri and attaches the query parameters code and scope.
Token Endpoint
After the user has interacted with the authorization endpoint and has landed on your redirect_uri, you can request an access token from the following URL:
https://prod.api.basf.com/security/internal/v1/oauth2/token
Request
You need to send the following fields in your POST request body as x-www-form-urlencoded data:
Name |
Description |
Example |
---|---|---|
client_id |
Your application’s Client ID |
c653ff6e-a86a-4531-8443-a8a4378dc2fb |
client_secret |
Your application’s Client Secret |
F2vC5iqfdMuLYltNuqJ… (truncated) |
grant_type |
The type of grant; must be “authorization_code”. |
authorization_code |
code |
The code received from the authorization endpoint. |
eyJhbGciOiJBMTI4S1ci… (truncated) |
redirect_uri |
The same redirect URI that you provided to the authorization endpoint. |
https://example.com/ |
CURL Example
curl https://prod.api.basf.com/security/internal/v1/oauth2/token \ -d "client_id=c653ff6e-a86a-4531-8443-a8a4378dc2fb" \ -d "client_secret=F2vC5iqfdMuLYltNuqJ…" \ -d "grant_type=authorization_code" \ -d "code=eyJhbGciOiJBMTI4S1ci…" \ -d "redirect_uri=https://example.com/"
Response
You receive a JSON-formatted response with the following fields:
Name |
Description |
Example |
---|---|---|
access_token |
The access token that you can use to make API requests. |
eyJhbGciOiJBMTI4S1ciLCJl… (truncated) |
token_type |
The access token’s type. Always equal to “bearer”. |
Bearer |
expires_in |
The number of seconds until the access token expires. |
43199 |
refresh_token |
The refresh token that you can use to retrieve a new access token after the previous one expired. Only some requests return this. Others omit the field. |
|
id_token |
If the openid scope was provided, the ID Token contains information about the identity of the user. Otherwise, the response omits this field. |
eyJ0eXAiOiJKV1QiLCJh… (truncated) |
scope |
The scope of the access token. This should be equivalent to the scope you sent to the authorization endpoint (except for openid, which is not included). |
apim |
JSON Example
{ "access_token": "eyJhbGciOiJBMTI4S1ciLCJl… ", "token_type": "bearer", "expires_in": 43199, "id_token": "eyJ0eXAiOiJKV1QiLCJh… ", "scope": "entprdatalakepermitappprod" }
Sending API Requests
To make an authorized API request, add an HTTP Authorization header with the word Bearer, followed by the access token.
(add curl example here)
OAuth with Client Certificates
The steps for OAuth with client certificates for functional users are the following:
- Send your certificate to the cookie endpoint and get an authentication cookie.
- With the authentication cookie, request an authorization code from the certificate authorization endpoint.
- With the authorization code, request an access token. This step is equivalent to the regular OAuth flow for individual users. You can refer to the token endpoint documentation above.
Cookie Endpoint
Configure your client certificate and make an HTTP GET request to the following URL:
https://federation.basf.com/nidp/app/login?id=CYT
Please note that the endpoint responds with a redirect and cookies, so you need to make sure that your HTTP client follows redirects and keeps the received cookies.
If you're testing the OAuth flow with Postman, follow these tips:
- Go to the global Postman settings, click the Certificates tab and add your client certificate. Postman also provides a guide for certificate setup.
- You can go to the Settings tab of your request and toggle "Automatically follow redirects" to ON.
Certificate Authorization Endpoint
The endpoint for this step is located at the following URL:
https://federation.basf.com/nidp/oauth/nam/authz
Please note that this is a different endpoint from the authorization endpoint used in the OAuth flow for apps with user context. While building the URL, you need to add the following query parameters:
Name |
Description |
Example |
Notes |
---|---|---|---|
response_type |
The type of response expected from the endpoint. Must be “code”. |
code |
We use the “code” grant type exclusively. |
redirect_uri |
URL to send the user to after authentication. |
https://example.com/ |
This parameter must match the URL provided during application registration. |
scope |
One or more scopes which you want to request. Separate multiple scopes with spaces. |
apim |
Applicable scopes as found in the respective API documentation. |
client_id |
Your application’s Client ID |
c653ff6e-a86a-4531-8443-a8a4378dc2fb |
The Client ID for your application can be found under My Apps in the developer portal. |
acr_values | Must be "Cert/Yes/Terminal". | Cert/Yes/Terminal | Fixed value. |
This endpoint also responds with a redirect, but as you're not in a browser, you typically do not want to follow to your redirect_url. Instead, you can read the code parameter from the redirect. Configure your HTTP client not to follow redirects.
If you're testing the OAuth flow with Postman, you can go to the Settings tab of your request and turn the "Automatically follow redirects" toggle mentioned above to OFF.
Then, read the Location header from the response. It should look like this:
{redirect_url}?code={code}
Extract the code and send it to the token endpoint, as explained in the previous section.